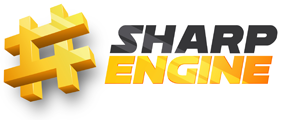 |
SceneNode Class |
SceneNode is a base abstract class that defines the common properties for all SceneNode objects.
SceneNodes objects define the 3D objects that are hierarchically organized by using
GroupNode object and define the 3D objects in the scene.
Inheritance Hierarchy Namespace: Ab4d.SharpEngine.SceneNodesAssembly: Ab4d.SharpEngine (in Ab4d.SharpEngine.dll) Version: 3.0.9208+3b2441d6a11f923f2600f40f4296bdc3d8b46035
Syntaxpublic abstract class SceneNode : InitializedSceneComponent,
IDisposable
The SceneNode type exposes the following members.
Constructors
Properties | Name | Description |
---|
 | DirtyFlags |
Gets the current dirty flags
|
 | IsHitTestVisible |
Gets or sets a Boolean that specifies if this SceneNode (and in case of GroupNode all its child SceneNodes) are visible to hit testing.
When false, then the object cannot be hit and the testing ray passes through this SceneNode and through its child SceneNodes.
Default value is true.
|
 | IsParentVisible |
True if parent is visible
|
 | IsVisible |
True if this SceneNode is actually visible (taking this and parent's Visibility into account).
|
 | IsWorldMatrixIdentity |
True if this SceneNode has no transformed and also its parents have no transformation.
|
 | Parent |
Gets the parent GroupNode of this SceneNode.
|
 | PreviousDirtyFlags |
Gets DirtyFlags value before the last Update method call.
The Update method the DirtyFlags value is reset to Unchanged.
This property is set in the CleanDirtyFlags method and preserves the DirtyFlags value before the last Update call.
|
 | Transform |
Gets or sets a transformation to this SceneNode.
|
 | Visibility |
Gets or sets a SceneNodeVisibility of this SceneNode.
If this SceneNode is a GroupNode, then setting this property to Hidden will also hide all the child SceneNodes.
To see if this SceneNode is actually visible (taking this and parent's Visibility into account) see the value of the IsVisible property.
Default value is Visible.
|
Top
Methods | Name | Description |
---|
  | CheckIfMaterialIsDisposed |
CheckIfMaterialIsDisposed
|
  | CheckIfMeshIsDisposed |
CheckIfMeshIsDisposed
|
  | CheckIfNonZeroPositive(Single, String) |
CheckIfNonZeroPositive
|
  | CheckIfNonZeroPositive(Vector2, String) |
CheckIfNonZeroPositive
|
  | CheckIfNonZeroPositive(Vector3, String) |
CheckIfNonZeroPositive
|
  | CheckIfNotSmallerThan(Int32, Int32, String) |
CheckIfNotSmallerThan
|
  | CheckIfNotSmallerThan(Single, Single, String) |
CheckIfNotSmallerThan
|
  | CheckIfPositiveOrZero(Single, String) |
CheckIfPositiveOrZero
|
  | CheckIfPositiveOrZero(Vector2, String) |
CheckIfPositiveOrZero
|
  | CheckIfPositiveOrZero(Vector3, String) |
CheckIfPositiveOrZero
|
 | CleanDirtyFlags |
Clean dirty flags on this and child nodes.
This should be called only after the frame was rendered.
|
 | ClearMeshDirtyFlag |
Clears the MeshDirty flag
|
 | CollectRenderingItems |
CollectRenderingItems
|
 | Dispose |
Dispose method disposes resources that are created by this SceneNode
(for example Mesh in PyramidModelNode or LineMaterial when LineNode is created with setting LineColor).
It does not dispose the resources that are created by the user and then set to properties in this SceneNode
(for example when a StandardMaterial is assigned to the Material property or Mesh is assigned to the MeshModelNode).
To also dispose the meshes and materials use the specialized dispose methods:
DisposeWithMaterial(Boolean),
DisposeWithMeshAndMaterial(Boolean, Boolean),
DisposeWithMaterial,
DisposeAllChildren(Boolean, Boolean, Boolean, Boolean),
DisposeWithAllChildren(Boolean, Boolean, Boolean, Boolean),
DisposeChildren(Int32, Int32, Boolean, Boolean, Boolean, Boolean).
|
 | Dispose(Boolean) |
Dispose
(Overrides ComponentBaseDispose(Boolean)) |
 | DisposeRenderingItems |
DisposeRenderingItems
|
 | GetInvertedWorldMatrix |
GetInvertedWorldMatrix method gets the inverted WorldMatrix into the matrix out parameter and returns true if matrix can be inverted; otherwise false is returned.
|
 | GetLocalBoundingBox |
Gets the BoundingBox of this SceneNode in local coordinates.
The BoundingBox is transformed by transformation on this SceneNode but not by parent's transformations.
This method always returns the value of localBoundingBox.
Derived classes can provide additional logic when updateIfDirty is true
(for example ModelNode can call UpdateMesh method when mesh is dirty;
GroupNode can update mesh on child SceneNodes, etc.).
|
 | GetOverviewText |
GetOverviewText adds string that writes details about this SceneNode into the specified StringBuilder.
|
 | NotifyAllParentSceneNodesChange |
Add the changeType to all parent SceneNodes.
This does not change this SceneNode's dirty flags.
|
 | NotifyChange |
Add the SceneNodeDirtyFlags to this SceneNode's DirtyFlags flags.
This methods also calls NotifyChange on parent DXScene.
|
 | OnIsVisibleChanged |
OnIsVisibleChanged is called when the value of IsVisible property is changed.
Overridden implementations should update the IsVisible property of the RenderingItem objects that were already added to RenderingLayers.
|
 | OnParentChanged |
OnParentChanged
|
 | OnTransformChanged |
OnTransformChanged is called when Transform or Parent's Transform is changed.
This method updates the WorldMatrix and sets WorldMatrixChanged dirty flags.
The method can be overridden to provide custom transformation handling.
|
 | OnUpdate |
OnUpdate
|
 | OnWorldMatrixChanged |
OnWorldMatrixChanged
|
 | ToString |
Returns a String that represents this instance.
(Overrides ObjectToString) |
 | Update |
Update method is called before every rendering and updates the graphics buffers and states objects based on the current value of properties in this SceneNode.
|
 | UpdateIsVisible |
UpdateIsVisible
|
 | UpdateLocalBoundingBox |
UpdateLocalBoundingBox
|
 | UpdateWorldBoundingBox |
UpdateWorldBoundingBox
|
 | UpdateWorldMatrix |
UpdateWorldMatrix
|
Top
Events | Name | Description |
---|
 | ParentChanged |
Occurs when Parent is changed.
Occurs also when this SceneNode is added to the SceneNodes tree for the first time or when it is removed from the SceneNodes tree (Parent is set to null).
This event does not occur when this SceneNode is disposed.
|
Top
Fields | Name | Description |
---|
 | localBoundingBox |
BoundingBox in local coordinates that can be get or set by derived classes.
The BoundingBox is transformed by transformation on this SceneNode but not by parent's transformations.
Its value can be get by calling GetLocalBoundingBox(Boolean) method.
|
 | stateFlags |
stateFlags is used to store multiple Boolean values in one int based enum.
This value should not be set directly but only through a property.
|
 | Tag |
Gets or sets an arbitrary object value that can be used to store custom information about this SceneNode.
|
 | WorldBoundingBox |
BoundingBox in world coordinates (transformed by parents transformations and transformation on this SceneNode).
This value is calculated by the engine and should not be changed by the user. It is a field and not a property for performance reasons.
|
 | WorldMatrix |
WorldMatrix defines the final world matrix for this SceneNode that is calculated from the parent's world matrix and this node's transformation matrix.
This value is calculated by the engine and should not be changed by the user.
|
Top
See Also