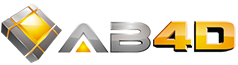 |
SimpleMeshT Class |
SimpleMesh class is used to create Index and Vertex buffers from a list of structs which type is defined by the type T.
For example the following struct SimpleMesh<PositionTexture> is used to define a mesh from a List of PositionTexture structs.
Inheritance Hierarchy Namespace: Ab3d.DirectXAssembly: Ab3d.DXEngine (in Ab3d.DXEngine.dll) Version: 7.1.9105.2048 (1.0.0.0)
Syntaxpublic class SimpleMesh<T> : MeshBase,
IDynamicVertexBufferCreator, IRayHitTestedObject, ITriangularMesh, IOctTreeMesh
where T : struct, new()
Type Parameters
- T
- struct that holds data for one vertex - for example Ab3d.DirectX.PositionNormalTexture
The SimpleMeshT type exposes the following members.
Constructors
Properties | Name | Description |
---|
 | ArrayStride |
Gets or sets an integer that specifies how many VertexBufferArray elements define one Vertex. Default value is 1. See remarks for more info.
|
 | CreateDynamicIndexBuffer |
Gets or sets a Boolean that specifies if the index buffer is created as a dynamic buffer.
This can provide slightly better performance for cases when index buffer is updated very often.
Default value is false.
|
 | CreateDynamicVertexBuffer |
Gets or sets a Boolean that specifies if the vertex buffer is created as a dynamic buffer.
This can provide slightly better performance for cases when vertex buffer is updated very often.
Default value is false.
|
 | IndexBufferArray |
Gets or sets an array that is used to define the index buffer
If this property is changed, then RecreateMesh(Boolean, Boolean, Boolean) method must be called.
|
 | OctTree |
Gets or sets an MeshOctTree that can be used for hit testing.
The MeshOctTree is automatically generated when number of positions in this mesh is bigger or equal to the value set in MeshPositionsCountForOctTreeGeneration.
|
 | TrianglesCount |
Gets the number of triangles that are used to define this mesh.
|
 | VertexBufferArray |
Gets or sets an array of T structs that define the vertex buffer.
If this property is changed, then RecreateMesh(Boolean, Boolean, Boolean) method must be called.
|
Top
Methods | Name | Description |
---|
 | CalculateBounds |
Calculates and updates Bounds from the specified VertexBufferArray.
The method can calculate bounds only when VertexBufferArray is of type PositionNormalTexture and PositionNormal.
|
 | CreateOctTree |
CreateOctTree returns a MeshOctTree that is created from the mesh defined in this SimpleMesh.
This method can be used when the type of the vertex buffer is PositionNormalTexture or Vector3 (only positions).
When the type of the vertex buffer is PositionNormal or PositionTexture, then vertex data are copied into positions array and an then OctTree is created.
Other types of vertex buffers are not supported and will generate an exception.
|
 | GetClosestHitResult |
GetClosestHitResult method returns a DXRayHitTestResult with the closest triangle hit by the specified ray.
When no triangle is hit, null is returned.
|
 | GetNextHitResult |
GetNextHitResult method continues hit testing from the previously returned hit test and returns a DXRayHitTestResult
with the next hit triangle (not necessary the next closest triangle) or null if no other triangle is hit.
This method can be used to get all hit results.
|
 | GetTrianglePositions |
GetTrianglePositions sets the 3 positions that define the triangle with the specified triangle index.
|
 | HitTest |
HitTest method test the triangles defined in this mesh for hitting with the specified ray.
When getOnlyNextHitTest is false, then the closest hit result is returned, else the next hit result is returned.
When no hit is found, null is returned.
|
 | RecreateMesh |
RecreateMesh disposes the existing VertexBuffers and IndexBuffer and recreates the buffers.
|
 | SortTrianglesByCameraDistance |
SortTrianglesByCameraDistance method sorts the triangle indices so that the triangles are sorted by camera distance -
triangles that are farther away are rendered first.
See remarks for more info.
|
Top
See Also