Transparency problem in WPF 3D
WPF 3D can show transparent 3D objects. But to show them correctly, the transparent
objects need to be defined after all the objects that are visible through them.
The following image shows the transparency problem in a simple 3D scene (because
the red boxes are defined after the semi-transparent GlassPlane they are not visible
through the GlassPlane. The order of objects is visible in the right):
To solve the problem, the GlassPlane needs to be moved after the non-transparent
objects. The following image is showing the correctly rendered scene.
For cases when the number of transparent objects is not big and if the transparent
objects are not positioned in 3D space one after another, the simple transparency
sorting is usually enough to produce correct rendering in WPF 3D.
But simply moving transparent objects after non-transparent objects is not always
enough. For example if transparent object A is visible through transparent object
B, than B must be defined after A. The problem in this case occurs when the camera
is rotated so that B is visible through A. Now B must be defined before A. To solve
this problem correctly, firstly the transparent objects must be moved after non-transparent
objects and than transparent objects must be sorted by their distance to the camera.
The sorting must be done after the camera is changed.
Partial solution with Ab3d.Reader3ds
Ab3d.Reader3ds can perform a simple transparency sorting by moving transparent
objects after non-transparent objects. Transparency sorting is performed if the
IsTransparencySortingEnabled is set to true (by default). It is also possible
to get the number of transparent objects by the TransparentObjectsCount property.
Ab3d.Reader3ds does not do any sorting by camera distance. For this the Ab3d.PowerToys
library is needed.
Full solution with Ab3d.PowerToys
With TransparencySorter class from Ab3d.PowerToys library it is possible to perform
simple transparency or to ByCameraDistance transparency
sorting where the transparent objects are sorted by their distance to the camera.
Simple transparency sorting (moving transparent objects after non-transparent objects) can be done with the following line:
Ab3d.Utilities.TransparencySorter.SimpleSort(myModelsGroup);
When sorting by camera distance is used, the TransparencySorter can also automatically
resort the objects when camera is changed. To optimize the sorting it is possible
to specify an angle that will tell how much the camera's direction must be changed
when the resorting is preformed.
To perform transparency sorting by camera distance the following lines of code can be used:
private TransparencySorter _transparencySorter;
private void StartTransparencySorting()
{
_transparencySorter = new TransparencySorter(_rootModel3DGroup, Camera1);
// Re-sort on every 10 degrees change
_transparencySorter.CameraAngleChange = 10;
// Do an initial sorting
_transparencySorter.Sort();
_transparencySorter.StartSortingOnCameraChanged();
}
private void StopTransparencySorting()
{
if (_transparencySorter != null)
_transparencySorter.StopSortingOnCameraChanged();
}
For more code samples see the samples that come with Ab3d.PowerToys library.
Recommendations
It is recommended to set IsTransparencySortingEnabled in Reader3ds to false. After
the 3ds file is read, check the TransparentObjectsCount to get the number of transparent objects.
If there are no transparent objects found, than no transparency sorting is needed.
If there are only a few transparent objects, than a simple transparency sorting
can be made with using Ab3d.Utilities.TransparencySorter.SimpleSort method.
If there are more transparent objects read or if there are only a few of them and
you want to be sure that they will be correctly sorted, create an instance of TransparencySorter
class and call StartSortingOnCameraChanged method to resort the transparent objects
when the camera is changed.
Samples
The following images show rendering of the objects before (left image) and after (right image) transparency sorting:
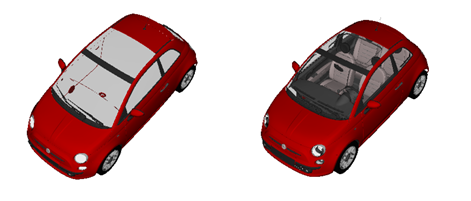
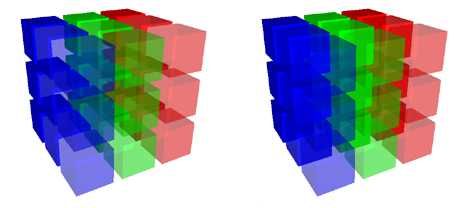
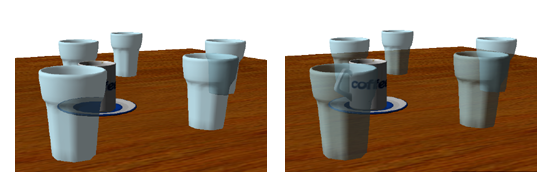
|