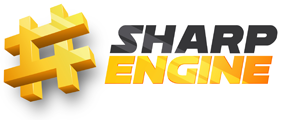 |
Camera Class |
Camera is a base camera that is used as a base class for all camera classes in SharpEngine except for
MatrixCamera.
Inheritance Hierarchy Namespace: Ab4d.SharpEngine.CamerasAssembly: Ab4d.SharpEngine (in Ab4d.SharpEngine.dll) Version: 3.0.9208+3b2441d6a11f923f2600f40f4296bdc3d8b46035
Syntaxpublic abstract class Camera : ICamera,
IPerspectiveCamera, IOrthographicCamera, IAutomaticNearFarPlaneCamera, ICameraWithLight, IRotatingCamera
The Camera type exposes the following members.
Constructors | Name | Description |
---|
 | Camera |
Protected constructor
|
Top
Properties | Name | Description |
---|
 | AspectRatio |
Gets or sets an aspect ratio (width divided by height) of the viewport that is showing the 3D scene.
|
 | AutomaticPlaneDistanceCalculationAdjustmentFactor |
Gets or sets a float that is multiplied by the automatically calculated near and far plane distance.
This makes the near plane slightly closer and far plane slightly farther away.
This prevents clipping the objects that are located very close to near and far planes.
Default value is 1.05 (5%).
|
 | CameraAnimation |
Gets the CameraAnimation class that can be used to animate the camera.
|
 | CameraLight |
Gets an instance of CameraLight that is created for this Camera.
This light is created based on the value of ShowCameraLight property.
|
 | FarPlaneDistance |
Gets or sets a value that specifies the distance from the camera of the camera's far clip plane.
By default NearPlaneDistance is automatically calculated from the 3D scene bounding box and camera position.
This can be disabled by setting IsAutomaticFarPlaneDistanceCalculation to false.
|
 | FieldOfView |
Gets or sets a value that represents the camera field of view in degrees.
By default this represents horizontal field of view, but this can be changed by setting the IsHorizontalFieldOfView property.
The property is used when the ProjectionType is set to Perspective and not for Orthographic projection type.
Default value is 45 degrees.
|
 | IsAutomaticFarPlaneDistanceCalculation |
Gets or sets a Boolean that specifies if is automatically calculated from the 3D scene bounding box and camera position.
This property is set to false, when the value of FarPlaneDistance is manually changed by the user.
Default value is true.
|
 | IsAutomaticNearPlaneDistanceCalculation |
Gets or sets a Boolean that specifies if is automatically calculated from the 3D scene bounding box and camera position.
This property is set to false, when the value of NearPlaneDistance is manually changed by the user.
Default value is true.
|
 | IsCameraChangedRaisedOnAutomaticPlaneDistanceCalculation |
Gets or sets a Boolean that specify if CameraChanged event is raised when
the NearPlaneDistance and FarPlaneDistance are
automatically calculated and then the SetPlaneDistances(Single, Single, Boolean) method is called.
Default value is false.
|
 | IsHorizontalFieldOfView |
Gets or sets a boolean that specifies if horizontal Field of View is used (as in WPF 3D) or vertical Field of view is used (false = as in DirectX).
Default value is true.
|
 | IsRotating |
Gets or sets a boolean that specifies if the camera is currently being animated.
|
 | MaxFarPlaneDistance |
When MaxFarPlaneDistance is set to any value that is not float.NaN and IsAutomaticFarPlaneDistanceCalculation is true, the calculated far plane distance is never bigger then the specified value.
This value must be bigger then MinNearPlaneDistance or its value is not used.
Default value is NaN.
|
 | MinNearPlaneDistance |
When MinNearPlaneDistance is set to any value that is not float.NaN and IsAutomaticNearPlaneDistanceCalculation is true, the calculated near plane distance is never smaller then the specified value.
Default value is NaN.
|
 | Name |
Gets or sets the name of the camera.
|
 | NearPlaneDistance |
Gets or sets a value that specifies the distance from the camera of the camera's near clip plane.
By default NearPlaneDistance is automatically calculated from the 3D scene bounding box and camera position.
This can be disabled by setting IsAutomaticNearPlaneDistanceCalculation to false.
|
 | ProjectionType |
Gets or sets the type of projection (perspective or orthographic). Default value is Perspective projection.
|
 | SceneView |
Gets the SceneView that this camera belong to. This property is set internally by the SceneView.
|
 | ShowCameraLight |
Gets or sets a value that defines if a CameraLight is created for this camera.
By default the value is set to Auto that means that the CameraLight is created when there are not other lights in the scene.
|
 | Version |
Gets the version number of this Camera. Version is increased each time the camera is changed.
This can be used to check if the camera was changed between two points in time.
Note: When camera is changed a lot of times, the Version value can overflow from positive to negative numbers (to prevent problems do not check for bigger value but for not equal value)
|
 | ViewWidth |
Gets or sets the width of the camera's view that is used only by orthographic camera (view height is get by dividing the ViewWidth by AspectRatio).
Default value is 100.
|
Top
Methods | Name | Description |
---|
  | CreatePerspectiveHorizontalFovRH |
PerspectiveFovRH calculates the matrix for the horizontal field of view for right handed coordinate system
|
  | DeserializeJson |
DeserializeJson deserialize the specified jsonString into a new instance of the camera.
The jsonString should be created by the SerializeToJson method.
If it is created manually, then the jsonString must start with the full type name of the camera.
|
 | GetCameraMatrices |
GetCameraMatrices method gets the camera's view and projection matrices.
When the optional parameter updateIfDirty is true (by default) then the Update(Boolean) method is called if the camera was changed after the last matrices were calculated.
|
 | GetCameraPlaneOrientation |
GetCameraPlaneOrientation gets plane's normal, width and height vectors that can be used to orient the plane 3D model so that it is aligned with the camera view.
|
 | GetCameraPosition |
Returns the current position of the camera.
|
 | GetInvertedViewMatrix |
GetInvertedViewMatrix method returns the inverted camera's view matrix.
The inverted view matrix can be used to revert the camera's rotation and its offset (to reset the offset, set M41, M42 and M43 to 0).
The calculated inverted matrix is cached until the camera is changed (the Matrix4x4.Invert is called only the first time this method is called).
|
 | GetLookDirection |
Returns the look direction of the camera.
|
 | GetUpDirection |
Returns the up direction of the camera.
|
 | GetViewMatrix |
GetViewMatrix method returns the camera's view matrix.
When the optional parameter updateIfDirty is true (by default) then the Update(Boolean) method is called if the camera was changed after the last matrices were calculated.
|
 | GetViewProjectionMatrix |
Gets a precalculated View * Projection matrix.
When the optional parameter updateIfDirty is true (by default) then the Update(Boolean) method is called if the camera was changed after the last matrices were calculated.
|
 | OnCameraChanged |
OnCameraChanged
|
 | OnCameraLightCreated |
OnCameraLightCreated
|
 | OnIsRotatingChanged |
OnIsRotatingChanged is called to fire IsRotatingChanged event.
|
 | OnSceneViewChanged |
OnSceneViewChanged
|
 | RotateCamera |
Rotates the camera for the specified heading and attitude.
|
 | SerializeToJson |
SerializeToJson serializes the specified camera into a json string.
The returned string starts with assembly qualified camera type name.
The serialized json can be converted to a camera by calling static DeserializeJson(String) method.
|
 | SetCameraMatrices |
SetCameraMatrices method can be called from a derived class to change the View and Projection matrix after the View and Projection matrices were calculated by the derived class.
|
 | SetPlaneDistances |
SetPlaneDistances method is used to set NearPlaneDistance and FarPlaneDistance
after the values are automatically calculated.
Bases on the value of IsCameraChangedRaisedOnAutomaticPlaneDistanceCalculation the CameraChanged event is raised.
|
 | StartRotation(Single, Single) |
StartRotation method immediately starts Heading and Attitude animation for this camera.
To slowly start the camera rotation and than accelerate the rotation, use the StartRotation(Single, Single, Single, FuncSingle, Single) method.
|
 | StartRotation(Single, Single, Single, FuncSingle, Single) |
StartRotation method slowly starts the camera rotation (animating Heading and Attitude properties) and than accelerates the rotation.
To immediately start rotation for this camera, use the StartRotation(Single, Single) method.
|
 | StopRotation |
StopRotation immediately stops the rotation animation of the camera.
|
 | StopRotation(Single, FuncSingle, Single) |
StopRotation slowly stops the rotation animation of the camera with preserving the rotation inertia.
|
 | Update |
Update method updates the camera matrices when needed (when there were any change in the camera properties) or when forceMatrixUpdate is set to true.
|
 | UpdateAnimations |
UpdateAnimations method updates the camera's rotation when it is animated.
|
 | UpdateCameraLight |
Updates the CameraLight based on the value of ShowCameraLight.
This method also disables all CameraLights that do not use this camera (for example when the same Scene is shown in multiple SceneViews and each camera defines its own CameraLight).
|
Top
Events | Name | Description |
---|
 | CameraChanged |
CameraChanged event is triggered when a property of this camera is changed.
|
 | CameraLightCreated |
CameraLightCreated event is triggered when a new CameraLight is created and assigned to the CameraLight property.
|
 | IsRotatingChanged |
IsRotatingChanged event is fired when the IsRotating is changed.
|
Top
Fields | Name | Description |
---|
 | boundingBoxCorners |
boundingBoxCorners (internally used to prevent allocating new array when bounding box corners are retrieved)
|
 | fitToViewActionWhenViewSizeIsValid |
fitToViewActionWhenViewSizeIsValid
|
 | isMatrixDirty |
isMatrixDirty is true after any property on camera has changed and signals that the View and Projection cameras need to be recalculated in the Update method.
|
Top
See Also