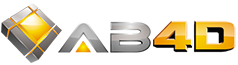 |
AnimationController Class |
Inheritance Hierarchy Namespace: Ab3d.AnimationAssembly: Ab3d.PowerToys (in Ab3d.PowerToys.dll) Version: 11.2.9104.2045
Syntaxpublic class AnimationController : ICompositionRenderingSubscriber
The AnimationController type exposes the following members.
Constructors
Properties | Name | Description |
---|
 | AnimationNodes |
Gets a list of AnimationNodeBase object that are animated by this AnimationController.
|
 | AnimationStartTime |
Gets a DateTime that specifies the start time of the animation (when the StartAnimation(Boolean) or StartAnimation(Double, Boolean)method was called).
If animation is not started a DateTime.MinValue is returned.
|
 | AutoRepeat |
Gets or set if the animation should automatically repeat itself or not.
Default value is false.
|
 | AutoReverse |
Gets or sets if animation should go backwards when coming to the last frame or should it start from beginning.
Default value is false.
|
 | AutoStopAnimation |
Gets or sets if animation is automatically stopped (calling StopAnimation method) when the animation is completed.
Animation can be completed only when AutoRepeat property is false.
In case AutoRepeat and AutoReverse are false the animation is completed when the LastFrameNumber is reached;
when AutoReverse is true, the animation is completed when the first frame is reached again (after reversing back from the last frame).
The property is used only only when StartAnimation(Boolean) or StartAnimation(Double, Boolean) method is called without parameters or with subscribeToRenderingEvent parameter set to true.
Default value is true.
|
 | CurrentFrameNumber |
Gets the frame number where this animation controller currently is - the same as frameNumber parameter in the last call to GoToFrame(Double) method.
|
 | FirstFrameNumber |
Gets the first defined frame number for this AnimationController.
This number is calculated each time with going though all AnimationNodes.
|
 | FramesPerSecond |
Gets or sets a double value that specifies for how many frames per second animation advances.
This does not change the rendering but the speed of animation. The frame numbers are not related to rendering frames but are defined in the KeyFrameBase and derived objects.
|
 | IsAnimating |
Gets a Boolean that specifies if animation is currently running (was started and is not paused).
|
 | IsAnimationPaused |
Gets a Boolean that specifies if animation was started and and is currently paused.
|
 | IsAnimationStarted |
Gets a Boolean that specifies if animation was started and is not yet stopped (also returns true if animation is paused).
|
 | LastFrameNumber |
Gets the last defined frame number for this AnimationController.
This number is calculated each time with going though all AnimationNodes.
|
Top
Methods | Name | Description |
---|
 | Dump |
Writes details about this AnimationNodeBase to the Visual Studio Output window.
This method calls the GetDumpString method to get details about each keyframe.
|
 | GetAnimationPauseTimeInSeconds |
Returns total elapsed time in seconds in which the animation was paused (time during PauseAnimation and ResumeAnimation method calls).
|
 | GetAnimationTimeInSeconds |
Returns time in seconds that elapsed from the start of the animation (time after calling StartAnimation(Boolean) or StartAnimation(Double, Boolean)). The pause time is not included in the returned time.
If animation was not started, 0 is returned.
To get the elapsed time to some specified time use the GetAnimationTimeInSeconds(DateTime) method that takes time as parameter.
|
 | GetAnimationTimeInSeconds(DateTime) |
Returns time in seconds that elapsed from the start of the animation to the time specified as a parameter to this method. The pause time is not included in the returned time.
When animation is started with startFrameNumber parameter, then the animation time is increased by the startFrameNumber divided by FramesPerSecond.
If animation was not started, 0 is returned.
|
 | GetDumpString |
GetDumpString virtual method can be overridden to provide detailed description of this object.
|
 | GetFrameNumber |
GetFrameNumber returns a double that specifies the current frame number (can be a fraction between two integer values).
The number is calculated based on the current animation time (get with calling GetAnimationTimeInSeconds method).
If the animation was not yet starter or first frame was not yet reached, then -1 us returned.
When animation has passed the last frame and the animation is not repeating, then the last frame number is returned.
|
 | GetFrameNumber(Double) |
GetFrameNumber returns a double that specifies the frame number at the specified animationTimeInSeconds (can be a fraction between two integer values).
If the animation was not yet starter or first frame was not yet reached, then -1 us returned.
When animation has passed the last frame and the animation is not repeating, then the last frame number is returned.
|
 | GoToFrame |
GoToFrame method updates the objects animated with this animation node based on the specified frame number.
The method calls GoToFrame(Double) method on all AnimationNodes objects.
|
 | OnAfterFrameUpdated |
OnAfterFrameUpdated fires AfterFrameUpdated event
|
 | OnAnimationCompleted |
OnAnimationCompleted fires AnimationCompleted event
|
 | OnAnimationStarted |
OnAnimationStarted fires AnimationStarted event
|
 | OnAnimationStopped |
OnAnimationStopped fires AnimationStopped event
|
 | OnBeforeFrameUpdated |
OnBeforeFrameUpdated fires BeforeFrameUpdated event
|
 | PauseAnimation |
PauseAnimation method pauses the animation. After pausing the animation, the call to GetFrameNumber method will return the frame number at the moment of pausing the animation.
To resume the animation call the ResumeAnimation method.
|
 | ResumeAnimation |
ResumeAnimation method resumes the animation after it was paused with PauseAnimation method.
|
 | StartAnimation(Boolean) |
StartAnimation method starts the animation in this AnimationController. If the animation was paused, this method continues the animation (is the same as ResumeAnimation method).
When the subscribeToRenderingEvent parameter is true (by default), then animation controller subscribes to the WPF's RenderingEvent
and automatically updates the animated objects by calling GetFrameNumber and GoToFrame(Double) methods.
If subscribeToRenderingEvent is false, user needs to manually call GetFrameNumber or other methods to update the animated objects.
|
 | StartAnimation(Double, Boolean) |
StartAnimation method starts the animation in this AnimationController. If the animation was paused, this method continues the animation (is the same as ResumeAnimation method).
When the subscribeToRenderingEvent parameter is true (by default), then animation controller subscribes to the WPF's RenderingEvent
and automatically updates the animated objects by calling GetFrameNumber and GoToFrame(Double) methods.
If subscribeToRenderingEvent is false, user needs to manually call GetFrameNumber or other methods to update the animated objects.
|
 | StopAnimation |
StopAnimation method stops the animation. After animation has been stopped, the call to GetFrameNumber method will return the first frame (index = 0).
|
Top
Events
Fields
See Also